Authorizing OAuth Apps
You can enable other users to authorize your OAuth App.
vivenu's OAuth implementation support the standard authorization code.
Caution: Never send your clientSecret
to the client. Send it only in server-to-server communication.
Web Application Flow
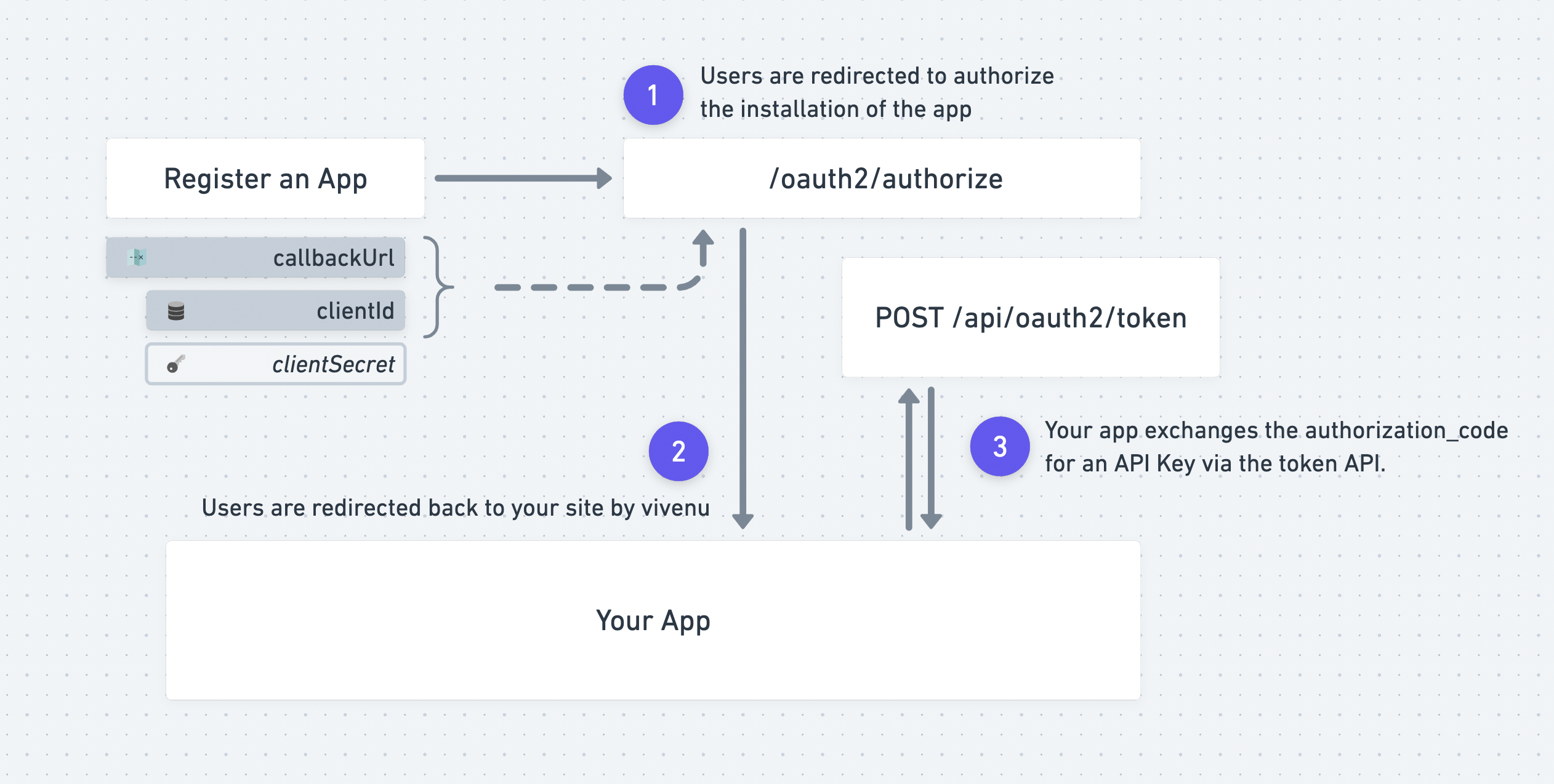
The web application flow to authorize users for your app is:
- Users are redirected to authorize the installation of the app
- Users are redirected back to your site by vivenu
- Your app exchanges the authorization_code for an API key via the token API.
- Optional: Redirect the user back to
https://dashboard.vivenu.com/apps/${CLIENT_ID}
If your app is installed through the vivenu marketplace you can skip step 1.
1. Request an app installation
GET https://dashboard.vivenu.com/oauth2/authorize
Parameters
Name | Type | Description |
---|---|---|
response_type | string | Required. The type of the response. Valid code |
client_id | string | Required. The client ID you received from vivenu when registered. |
redirect_uri | string | Required. The URL in your application where users will be sent after authorization. |
state | string | An unguessable random string. It is used to protect against cross-site request forgery attacks. If the installation request comes from the vivenu marketplace, we will create a signed state which you can verify. See below for more details |
2. Users are redirected back to your site by vivenu.
Caution: Users can be redirected to your site, even when they want to configure the App. It is not guaranteed, that only new users come to this URL.
If the user authorizes your app installation, vivenu redirects back to your site with a temporary code in a
code
parameter as well as the state you provided in the previous step in a state
parameter.
The temporary code will expire after 15 minutes.
If an error occured in this processs the user will also be redirected to your site.
If the states don't match, then a third party created the request, and you should abort the process.
For apps installed through the vivenu marketplace, you can verify the validity of the request as described here.
GET https://your-site.com/oauth2/callback
Success - Query Parameters
Name | Type | Description |
---|---|---|
code | string | This code can be exchanged for an API key |
state | string | It is used to protect against cross-site request forgery attacks. |
Error - Query Parameters
Name | Type | Description |
---|---|---|
error | string | An error code |
error_description | string | Description about the occured error |
3. Your app exchanges the authorization_code for an API key via the token API.
To be able to authenticate as a user you need to exchange the code
parameter received from the redirect for an API key:
POST https://apps.vivenu.com/api/oauth2/token
Parameters
Name | Type | Description |
---|---|---|
grant_type | string | Required. The grant type. Valid authorization_code |
client_id | string | Required. The client ID you received from vivenu for your OAuth App. |
client_secret | string | Required. The client secret you received from vivenu for your OAuth App. |
code | string | Required. The code you received as a response to Step 1. |
redirect_uri | string | Required. The URL in your application where users are sent after authorization. |
Response
{
"access_token": "key_app_2d5f3b351d7da30644738598dc87d5c0b3d9bcedbae66f"
}
The returned access_token
can be used as an API key as described on the introduction page.
Verify state query param
When an app installation request comes from the vivenu marketplace you can check the validity of the request by verifying the state query param of the request.
import { createHmac } from "crypto";
const state = createHmac("sha256", CLIENT_SECRET)
.update(request.query.code)
.digest("base64url");
if (state != request.query.state) {
throw new Error("State mismatch");
}
Get meta data for API key
If you need the installation metadata to update the installation status or save a state for your application, you can invoke the metadata API with your provided API key by including it as an authorization header to retrieve the necessary information.
GET https://apps.vivenu.com/api/oauth2/meta
Response
{
"installationId": "5de40f5b-ab20-4c62-af15-4c7774a42e9a"
}
Further reading
With the installationId
received from the previous section you can manage your application logic by integrating it to the vivenu application.